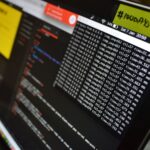
Error Handling and Exception Handling in Swift
March 19, 2024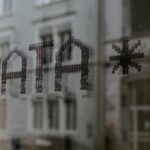
Swift File Handling and Input/Output Operations
March 19, 2024In the world of Swift programming, collections and data structures play a vital role in organizing and manipulating data efficiently. From arrays and dictionaries to sets and queues, these powerful tools allow developers to store and retrieve data in a structured manner. In this article, we will explore the various collection types available in Swift and delve into the intricacies of working with them. Whether you are a beginner or an experienced Swift programmer, understanding and utilizing collections and data structures effectively is essential for writing clean, optimized code. We will discuss the benefits and use cases of different collection types, along with common operations and best practices for working with them. By the end of this article, you will have a solid understanding of Swift collections and data structures, enabling you to leverage their power in your own projects. So let’s dive in and explore the world of Swift collections, and learn how to harness their potential for building robust and efficient applications.
Swift collections and data structures
Table of Contents
Arrays in Swift
Swift arrays are one of the most commonly used data structures. They are ordered collections of values. The same value can appear in an array multiple times at different positions.
An array’s values are always of the same type, which makes the array a homogeneous data structure. For example, an array can hold a list of integers, strings, or any other type of object, but it cannot hold a mixture.
Swift arrays offer various functionalities such as appending, inserting, and removing items, as well as querying the number of items and accessing items by index. They are extremely flexible and can be resized dynamically, which means you can add and remove items as needed.
Dictionaries in Swift
Swift dictionaries, like arrays, are collections. However, they differ from arrays in that they store associations between keys of the same type and values of the same type.
A dictionary’s keys are unique and act as an identifier for the values they relate to. This makes dictionaries ideal for storing values that are uniquely associated with a particular key, such as the names and ages of a group of people.
Working with dictionaries involves adding, removing, and modifying items, as well as querying for the existence of a key or value. Because dictionaries are unordered collections, the order of their elements is not fixed and cannot be relied upon.
Sets in Swift
Sets in Swift are a type of collection that store unique values of the same type in no particular order. They are similar to arrays and dictionaries but have their distinct features.
A set’s primary advantage is its ability to efficiently check whether it contains a particular item. This is due to its implementation, which uses a technique called hashing to store and retrieve values.
Because sets store unique values, they are the perfect tool for modelling collections of items where each item only appears once, such as a list of unique names or IDs.
Tuples in Swift
Tuples are a simple and flexible way to group related values together. Unlike arrays or sets, tuples can store values of different types.
Tuples are particularly useful when you want to return multiple values from a function. They allow you to package these values together into a single compound value.
However, tuples are not suited for complex data structures. They are best used for temporary groupings of related values, not for large collections or data that requires methods and capabilities.
Linked Lists in Swift
Linked lists are a more advanced type of data structure in Swift. A linked list is a sequence of data elements, which are connected together via links. Each data element contains a connection to another data element in form of a pointer.
Linked lists are ideal for creating dynamic data structures, as they can easily be resized. This contrasts with arrays, which have a fixed size once created.
However, linked lists have a significant downside: accessing elements is not as straightforward as in arrays or dictionaries, as you have to traverse the list from the start to find the element you’re after.
Stacks and Queues in Swift
Stacks and queues are two more advanced types of collections in Swift. Both are used to store multiple values, but they differ in how elements are added and removed.
A stack follows the Last-In-First-Out (LIFO) rule. This means that the last element added to the stack is the first one to be removed. It’s akin to a stack of plates: the last plate added to the top of the stack is the first one you’d take off.
A queue, on the other hand, follows the First-In-First-Out (FIFO) rule. The first element added is the first one to be removed. It’s similar to a queue of people: the first person in line is the first one to be served.
Trees and Graphs in Swift
Trees and graphs represent more complex data structures in Swift. They are used to represent hierarchical relationships between objects.
A tree consists of nodes, where each node has a value and a list of references to other nodes (its children). The top node in a tree is called the root, and nodes with no children are called leaves.
A graph, on the other hand, is a collection of nodes (or vertices) and edges. Each edge connects two vertices. Unlike trees, graphs can have cycles, which means it’s possible to start at one vertex and traverse the graph to end up at the same vertex.
Choosing the Right Data Structure in Swift
Choosing the right data structure in Swift depends on the specifics of the task you’re tackling. You need to consider factors such as the type of data you’re dealing with, the operations you need to perform, and the performance characteristics of the data structure.
For example, if you need to store a list of items and the order matters, an array would be a good choice. If you need to quickly check whether a collection contains a certain item, a set would serve you well.
For more complex tasks, such as representing hierarchical relationships, you might need to use trees or graphs. The key is to understand the strengths and weaknesses of each data structure and choose the one that best fits your needs.
Conclusion and Further Resources
Understanding Swift collections and data structures is crucial for writing efficient and effective code. This article has covered the basics, but there’s much more to learn.
There are many resources available to help you deepen your understanding of these topics. The Swift Programming Guide from Apple is a great place to start. It provides detailed explanations and examples of all the collection types and data structures we’ve discussed.
With practice and study, you’ll learn to use these tools to solve complex problems and write better, more efficient code. Keep exploring, keep learning, and keep coding in Swift!