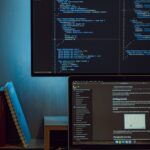
Basics of Swift Syntax and Data Types in Swift
March 18, 2024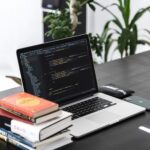
Unveiling the Power of Object-Oriented Programming in Swift: Crafting Structured and Scalable Code
March 18, 2024In the world of Swift programming, control flow and decision-making are like the building blocks of a solid foundation. They shape the path your code takes, allowing you to dictate the behavior of your program. Whether it’s executing certain lines of code based on a specific condition or repeating a task until a certain condition is met, understanding control flow and decision-making is essential for any Swift developer. In this article, we’ll dive deep into the concepts of control flow and decision-making in Swift, exploring the various control structures and syntax that Swift offers. We’ll cover everything from if statements and switch statements to loops and conditional expressions. By the end of this article, you’ll have a clear understanding of how to effectively control the flow of your Swift programs and make informed decisions to achieve the desired outcomes. So, if you’re ready to elevate your Swift programming skills and gain mastery over control flow and decision-making, let’s get started!
Control Flow Statements in Swift
Control flow in Swift, like any other programming language, is all about managing the order in which your code executes. Swift offers a variety of control flow statements including for
and while
loops, if
and switch
statements, and control transfer statements like break
and continue
.
Understanding these control flow statements is crucial as they allow you to handle complex problems with ease. Without them, your code would run from beginning to end without any deviation, which is hardly ever what you want. These statements allow you to add logic to your code, enabling it to react to different situations and conditions dynamically.
Let’s take the if
statement, for example. It’s used to execute a block of code only if a certain condition is met. If the condition isn’t met, the block of code is skipped, and the program continues on. This is a fundamental aspect of control flow in Swift and forms the basis of decision-making in your programs.
Conditional Statements in Swift (if, else-if, else)
Swift offers a variety of conditional statements, including if
, else-if
, and else
. These statements help you control the execution of your code based on certain conditions.
An if
statement checks a condition: if the condition is true, the block of code within the if
statement is executed. If the condition is false, it skips the block of code. The else-if
statement can be added after an if
statement to check for additional conditions. The else
statement, on the other hand, executes a block of code if none of the conditions in the preceding if
or else-if
statements are true.
These conditional statements are an essential part of Swift programming. By using them effectively, you can create complex logic in your programs, enabling them to handle a variety of situations and react accordingly.
Switch Statements in Swift
In addition to the if
, else-if
, and else
conditional statements, Swift also offers a switch
statement for decision-making. The switch
statement is a powerful tool that gives your programs the ability to evaluate multiple conditions and execute different blocks of code based on the outcome.
The switch
statement in Swift is quite versatile compared to other programming languages. It supports a variety of data types and allows for complex pattern matching. This makes it a powerful tool for controlling the flow of your code.
Using the switch
statement effectively can help you write cleaner, more organized code. It’s a great way to handle complex decision-making scenarios where you need to evaluate multiple conditions.
Looping Statements in Swift (for, while, repeat-while)
Looping, in programming, is the concept of repeating a block of code until a specific condition is met. Swift offers three types of loops: for
loops, while
loops, and repeat-while
loops.
A for
loop repeats a block of code a specific number of times. A while
loop, on the other hand, repeats a block of code as long as a certain condition is true. The repeat-while
loop, similar to a while
loop, also repeats a block of code based on a condition but guarantees that the loop will be executed at least once.
Understanding these loops and using them effectively is a key aspect of controlling the flow of your Swift programs. They allow you to perform repetitive tasks efficiently, making your code more concise and easier to read.
Using Control Flow and Decision-Making in Swift Functions
Functions in Swift are self-contained chunks of code that perform a specific task. You can use control flow and decision-making statements within these functions to dictate how they execute.
For instance, you can use an if
statement in a function to execute different blocks of code based on the input parameters. Similarly, you can use a for
loop in a function to perform a task repeatedly. By combining these control flow and decision-making tools within functions, you can create highly versatile and reusable code.
Understanding how to effectively use control flow and decision-making in Swift functions is a crucial part of becoming a proficient Swift developer. It allows you to write functions that are dynamic, flexible, and capable of handling a variety of situations.
Best Practices for Using Control Flow and Decision-Making in Swift
When using control flow and decision-making in Swift, there are several best practices to keep in mind. These best practices ensure your code is efficient, readable, and maintainable.
Firstly, always try to keep your functions and loops as simple as possible. Complex control flow can make your code difficult to read and understand. Secondly, use descriptive variable and function names to make your code self-documenting. This will make it easier for others (and future you) to understand your code.
Also, don’t forget to handle all possible cases in your switch
statements, and use break
and continue
statements judiciously in your loops. These practices will help you avoid common pitfalls and bugs in your code.
Debugging Control Flow and Decision-Making Issues in Swift
Sometimes, even with the best practices in place, you might encounter issues with control flow and decision-making in your Swift code. Debugging these issues can be a challenging task, but Swift offers several tools that can help.
The Swift debugger is a powerful tool that allows you to step through your code line by line, inspect variables, and watch expressions. By using the debugger effectively, you can identify issues in your control flow and find the root cause of any unexpected behavior.
Another valuable tool is Swift’s error handling mechanism. By using try
, catch
, and throw
, you can handle errors in your code effectively, making it more robust and reliable.
Resources for Learning More About Control Flow and Decision-Making in Swift
If you want to delve deeper into control flow and decision-making in Swift, there are many resources available. The Swift Programming Language Guide by Apple is a comprehensive resource that covers all aspects of Swift, including control flow and decision-making.
In addition, there are several online platforms like Udemy, Coursera, and Codecademy that offer Swift programming courses, where you can learn more about control flow and decision-making in a structured manner.
Also, don’t forget to join Swift communities on platforms like Stack Overflow and GitHub. They are great places to learn from experienced Swift developers and get help with any issues you might encounter.
Conclusion
Control flow and decision-making are fundamental aspects of Swift programming. They allow you to dictate the behavior of your programs, making them dynamic and capable of handling a variety of situations.
By understanding and using control flow statements, conditional statements, loops, and decision-making tools effectively, you can become a proficient Swift developer. So, keep practicing, keep learning, and don’t be afraid to dive deep into these concepts!