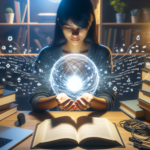
Start Your Coding Journey: Easy-to-Follow Swift Programming Tutorials
March 15, 2024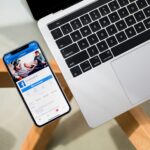
Building Your First iOS App using Swift
March 16, 2024Welcome to the exciting world of Swift! Whether you’re a seasoned programmer or just starting out, this article will help you get up to speed on this powerful and intuitive programming language. With its clean syntax and extensive features, Swift has become the go-to choice for building iOS, macOS, and watchOS applications.
In this comprehensive guide, we’ll break down the basics of Swift and walk you through the process of setting up your development environment. From there, we’ll dive into the fundamentals, exploring variables, loops, conditionals, and more. We’ll also introduce you to Swift’s object-oriented programming concepts, such as classes, inheritance, and protocols.
As we progress, we’ll cover more advanced topics like error handling, optionals, and closures. Additionally, we’ll discuss best practices and provide tips and tricks to help you write clean and efficient Swift code.
Getting Started with Swift
So, whether you’re looking to build your first iOS app or enhance your existing programming skills, this article is your go-to resource for getting started with Swift. Let’s dive in and unlock the potential of this incredible language.
Advantages of using Swift
Swift is not just a language; it’s an ecosystem designed with the developer in mind. It is a powerful language that is easy to learn and fun to use. Its simplicity and readability make it an excellent choice for beginners, while its depth and flexibility appeal to seasoned developers.
Moreover, Swift is a statically typed language. This means that the type of every variable is checked at compile-time, leading to fewer crashes and bugs in your code. Swift also supports type inference, allowing you to write less code while maintaining type safety.
Another significant advantage of Swift is its speed. Swift is fast – really fast. It’s optimized for performance and can compete with languages like C++ for most tasks. This makes Swift a good choice for data-intensive apps or those that require complex computations.
Swift vs. Objective-C: Key differences
While Objective-C had its day, Swift is undeniably the future of iOS development. The key differences between the two lie in the syntax, safety, and modern programming paradigms.
Swift’s syntax is cleaner, more streamlined, and more expressive than Objective-C’s. Objective-C’s syntax is a mix of Smalltalk’s messaging syntax and C’s procedural syntax, which can be confusing for newcomers. On the other hand, Swift’s syntax is easy to read and write, making it more accessible.
Safety is another area where Swift shines. Swift introduces optional types, which handle the absence of a value. In Objective-C, it’s common to have null pointer exceptions, but Swift’s optionals make such exceptions a thing of the past.
Finally, Swift is a more modern language than Objective-C. It has features like closures, generics, and tuples, which Objective-C lacks. Swift also supports functional programming patterns, which can lead to more concise and readable code.
Setting up the Swift development environment
Before we delve into Swift’s syntax and features, we need to set up our development environment. For macOS users, Apple provides an integrated development environment (IDE) called Xcode, which includes a Swift compiler, debugger, and a suite of powerful tools for building iOS and macOS apps.
To install Xcode, you’ll need an Apple ID. Head over to the Mac App Store, search for Xcode, and click on the “Get” button. Once the installation is complete, you’re ready to start coding in Swift.
For those on Windows or Linux, setting up a Swift development environment can be a bit challenging, as Xcode is not available on these platforms. However, there are alternatives like using a virtual machine or a cloud-based IDE like Repl.it or IBM’s Swift Sandbox.
Basic syntax and data types in Swift
Now that we’ve set up our development environment, let’s explore Swift’s basic syntax and data types. Swift’s syntax is clean, elegant, and easy to understand. It’s designed to make your code more readable and less prone to errors.
Swift supports most of the standard data types you’d expect, including integers, floats, booleans, and strings. It also introduces a few new ones, like the Optional
type, which represents a value that may or may not exist.
Swift also includes powerful collection types like arrays, sets, and dictionaries. These are used to store collections of values, and Swift provides many built-in functions to manipulate them.
Control flow and decision-making in Swift
Swift offers several control flow structures, including if
, guard
, switch
, and for-in
loops. These structures allow you to control the flow of execution in your program based on certain conditions or ranges.
Swift’s if
and guard
statements are used for decision-making. They evaluate a condition and execute a block of code based on whether that condition is true or false. The switch
statement in Swift is much more powerful than in other languages, supporting a variety of data types and complex pattern matching.
Swift also provides various loop structures for iteration, including for-in
, while
, and repeat-while
loops. These loops allow you to repeat a block of code a certain number of times or until a specific condition is met.
Functions and closures in Swift
Functions are fundamental to any programming language, and Swift is no exception. Functions in Swift are first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
Swift also introduces closures, which are self-contained, reusable blocks of code. Closures can capture and store references to variables and constants from the surrounding context, making them incredibly flexible and powerful.
Swift’s function and closure syntax is clean and concise, promoting code that’s easy to read and maintain. This is part of what makes Swift such a joy to work with.
Object-oriented programming in Swift
Swift is a multi-paradigm language, supporting both procedural and object-oriented programming styles. It provides all the key features of object-oriented programming (OOP), including classes, inheritance, and polymorphism.
In Swift, classes are blueprints for creating objects. They encapsulate data and functionality, promoting modularity and reusability. Swift also supports inheritance, allowing one class to inherit the properties and methods of another.
Swift introduces protocols, which define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Protocols are a powerful feature of Swift, providing a way to write more flexible and reusable code.
Error handling and debugging in Swift
No matter how experienced you are as a developer, errors are inevitable. Swift provides robust error handling mechanisms, allowing you to catch and handle errors gracefully.
Swift uses a combination of optional types and error propagation for error handling. This combination enables you to write safer code and recover from unexpected or erroneous conditions gracefully.
Debugging is another critical aspect of software development. Xcode provides a powerful suite of debugging tools, including breakpoints, a console for logging, and a view debugger for inspecting your app’s UI.
Conclusion and next steps in learning Swift
That wraps up our introduction to Swift! By now, you should have a solid understanding of Swift’s syntax, features, and the benefits it offers. You’ve also learned how to set up your development environment and got a taste of Swift’s object-oriented programming capabilities.
As your next steps, consider building a simple app in Swift. There’s no better way to learn than by doing. You could also explore Swift’s extensive documentation to deepen your understanding of the language.
Remember, becoming proficient in any programming language takes time and practice. Don’t be discouraged if you don’t understand everything at first. Keep coding, keep learning, and most importantly, have fun!