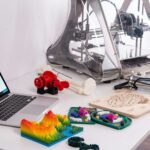
Swift Collections and Data Structures
March 19, 2024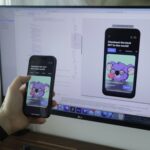
Introduction to SwiftUI for Building User Interfaces in Swift
March 21, 2024Are you a Swift developer looking to enhance your understanding of file handling and input/output operations in the language? Look no further! In this comprehensive article, we delve into the intricacies of Swift file handling, equipping you with the knowledge and skills needed to effectively manage files and perform input/output operations. Swift provides a robust set of APIs and tools that make working with files a breeze. Whether you’re reading from or writing to files, manipulating file metadata, or performing advanced file operations, you’ll find everything you need to know here. From understanding the different file paths to mastering file I/O techniques, we cover it all. We’ll explore how to open, close, and delete files, as well as how to read and write data to and from files using various approaches. By the end of this article, you’ll have a solid grasp of Swift file handling and input/output operations, enabling you to efficiently work with files in your projects. So let’s get started and dive into the world of Swift file handling!
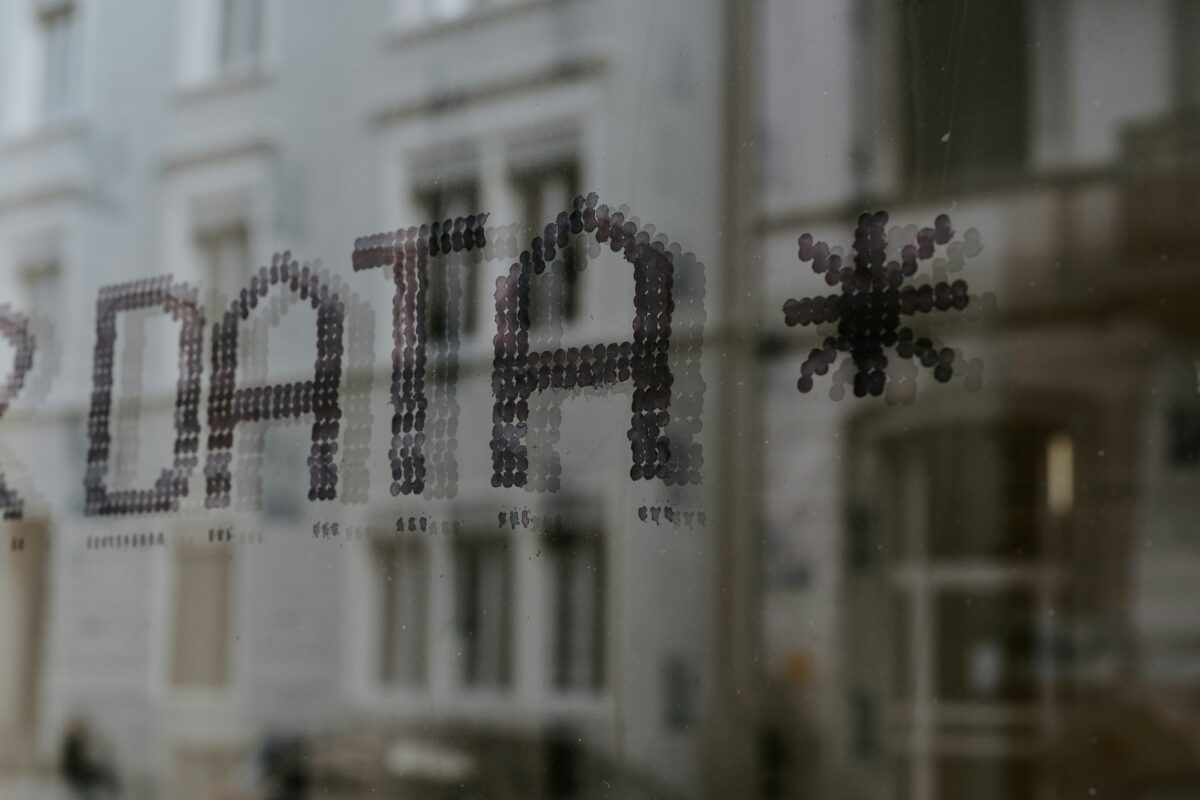
Swift File Handling and Input/Output Operations
Table of Contents
Different types of files and their purposes
Swift supports a wide range of file types, each with its unique purpose. Text files, for instance, are typically used for storing and retrieving textual data. They are simple to handle and can be read from or written to with ease. They are often used in tasks such as logging system events or storing configuration settings.
Binary files, on the other hand, are used for storing non-textual data such as images, audio files, and executable code. They hold data in a format that is not human-readable, but they offer the advantage of compact storage and quick access. Binary files are commonly used in applications that need to manage large data sets or perform complex data manipulations.
Lastly, we have JSON and XML files. These file types are used to store structured data in a human-readable format. They provide a standardized way to encode data structures like lists, arrays, and objects, making them ideal for tasks like data interchange between systems.
Reading data from a file in Swift
Reading data from a file in Swift is a straightforward process. Swift provides the readLine()
function, which reads a line of input from a text file. This function returns an optional string representing the line that was read, or nil if the end of the file has been reached.
Here is an example of how you can use this function to read data from a file:
“`swift import Foundation
let fileURL = URL(fileURLWithPath: “path/to/your/file.txt”) do { let fileContents = try String(contentsOf: fileURL, encoding: .utf8) let lines = fileContents.split(whereSeparator: .isNewline) for line in lines { print(line) } } catch { print(“Error: (error)”) } “` This code opens the file at the specified URL, reads its contents as a string, and then splits the string into lines. Each line is then printed to the console.
Writing data to a file in Swift
Writing data to a file in Swift is just as straightforward. You can use the write(to:atomically:encoding:)
function of the String
class to write a string to a file. Here’s an example:
“`swift import Foundation
let fileURL = URL(fileURLWithPath: “path/to/your/file.txt”) let stringToWrite = “Hello, Swift!” do { try stringToWrite.write(to: fileURL, atomically: true, encoding: .utf8) } catch { print(“Error: (error)”) } “`
In this example, the string “Hello, Swift!” is written to the file at the specified URL. If the file does not exist, it is created. If it does exist, its contents are replaced with the new string.
Handling errors in file operations
When performing file operations in Swift, it’s crucial to handle potential errors. Swift uses a system of error handling that involves throwing, catching, and propagating errors. Errors thrown from functions are propagated up the call stack until they are caught by a catch
clause.
In the previous examples, we’ve used a do-catch
statement to handle errors thrown by the readLine()
and write(to:atomically:encoding:)
functions. If an error is thrown, it’s caught and handled by printing an error message to the console.
This error handling mechanism makes Swift code safe and robust. By handling errors, we can ensure that our programs continue to run even in the face of unexpected situations.
File management and organization in Swift
Swift provides powerful tools for file management and organization. The FileManager
class, for instance, provides methods for creating, reading, and writing files and directories. It also provides methods for moving, copying, and deleting files, among other tasks.
Here’s an example of how you can use the FileManager
class to create a directory:
“`swift import Foundation
let fileManager = FileManager.default let directoryURL = URL(fileURLWithPath: “path/to/your/directory”, isDirectory: true)
do { try fileManager.createDirectory(at: directoryURL, withIntermediateDirectories: true) } catch { print(“Error: (error)”) } “`
In this example, a new directory is created at the specified URL. If any intermediate directories in the specified path don’t exist, they are also created.
Working with directories and file paths
Working with directories and file paths in Swift involves using the URL
and FileManager
classes. The URL
class provides methods for manipulating URLs, while the FileManager
class provides methods for interacting with the file system.
For instance, you can use the URL
class to extract the components of a file path, like this:
“`swift import Foundation
let fileURL = URL(fileURLWithPath: “path/to/your/file.txt”) print(fileURL.pathComponents) // prints: [“path”, “to”, “your”, “file.txt”] `` In this example, the
pathComponentsproperty of the
URL` class is used to extract the components of the file path.
Advanced file handling techniques in Swift
Swift offers several advanced techniques for file handling. For instance, you can read and write data from and to files asynchronously using the DispatchQueue
class. This allows you to perform file operations without blocking the main thread, which can improve the performance of your applications.
Here’s an example of how you can use the DispatchQueue
class to write data to a file asynchronously:
“`swift import Foundation
let fileURL = URL(fileURLWithPath: “path/to/your/file.txt”) let stringToWrite = “Hello, Swift!”
DispatchQueue.global().async { do { try stringToWrite.write(to: fileURL, atomically: true, encoding: .utf8) } catch { print(“Error: (error)”) } } `` In this example, the
write(to:atomically:encoding:)` function is called within a closure that’s dispatched to a global queue for execution in the background.
Best practices for efficient file handling in Swift
When working with files in Swift, it’s important to follow certain best practices to ensure efficient file handling. Here are some tips:
- Always handle errors: As we’ve seen, Swift provides a robust error handling mechanism. Always use it to handle potential errors when performing file operations.
- Use appropriate file types: Choose the right file type for the task at hand. For instance, use text files for storing textual data and binary files for storing non-textual data.
- Use asynchronous file operations: Whenever possible, perform file operations asynchronously. This can significantly improve the performance of your applications.
- Keep your file system organized: Use the tools provided by Swift to keep your file system organized. Create directories to group related files together and use descriptive names for your files and directories.
Conclusion and final thoughts
In this article, we’ve explored the intricacies of Swift file handling and input/output operations. We’ve covered everything from understanding different file types to mastering file I/O techniques.
By now, you should have a solid understanding of how to effectively manage files and perform input/output operations in Swift. Whether you’re reading from or writing to files, manipulating file metadata, or performing advanced file operations, you now have the knowledge and skills required.
So go ahead and put these concepts into practice. Happy Swift coding!