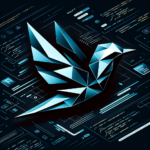
Getting Started with Swift
March 16, 2024Swift programming language, developed by Apple Inc., is a multi-paradigm, compiled language designed for iOS, macOS, watchOS, and tvOS development. It aims to overcome the challenges of Objective-C, supporting functional, imperative, and block-structured programming styles. Swift’s safety, precision, and speed are notable, alongside its compatibility with C, Objective-C, C++, allowing them to run within the same program. It enforces exclusive access to memory, enhancing program safety and performance [1] [2].
Key Features of Swift:
- Type Safety: Swift ensures that code interacts with the intended types, reducing errors [3].
- Memory Management: Utilizes automatic reference counting (ARC) for efficient memory usage without manual coding [5].
- Interoperability: Swift works seamlessly with existing Objective-C code, facilitating the use of vast libraries and codebases [4].
- Open Source: Since its open-sourcing in 2015, Swift has welcomed contributions from a broad developer community, supporting platforms beyond Apple’s ecosystem, including Linux and Windows [4].
Swift’s adoption of ideas from languages like Objective-C, Rust, Haskell, and Python contributes to its modern syntax and features, making it intuitive and powerful. It offers advanced types like tuples and optionals, and strong collection types such as Array, Set, and Dictionary for robust data handling. The playground feature allows for immediate visualization of outcomes, encouraging experimentation [3]. Swift’s versatility extends from app development to server-side programming, supported by a vibrant open-source community and continuous enhancements, making it an excellent choice for beginners and experienced developers alike [7].
Getting Started with Swift
To embark on your journey with Swift programming, it’s essential to familiarize yourself with the basics and set up your environment correctly. Here’s how to get started:
- Install Xcode: The primary tool for Swift development is Xcode, available for free on the Mac App Store. Xcode integrates all the tools needed to design, develop, and debug Swift applications [13].
- Explore Swift Playgrounds: For an interactive learning experience, Swift Playgrounds on iPad and Mac offers lessons and challenges that introduce core coding concepts in Swift. It’s a fun and engaging way to start coding without prior experience [12].
- Understand the Basics:
- Variables: Learn to store and manage data using variables (
var
) and constants (let
). Variables can hold various data types like integers and strings and can be reassigned new values [13]. - Constants: Unlike variables, constants are declared with the
let
keyword and cannot be reassigned. Understanding the difference and usage of variables and constants is crucial in Swift programming [13].
- Variables: Learn to store and manage data using variables (
- Begin Coding:
- First Steps: After installing Xcode, start with simple exercises like declaring variables, creating constants, and performing basic operations. Use the assignment operator (
=
) to assign values to your variables [13]. - Practice in Playgrounds: Utilize Xcode’s playgrounds for a hands-on approach to learning Swift. It allows for immediate feedback and experimentation with code [14].
- First Steps: After installing Xcode, start with simple exercises like declaring variables, creating constants, and performing basic operations. Use the assignment operator (
- Leverage Resources:
- Verify Installation: Before diving deep into coding, ensure Swift is correctly installed on your system. You can do this by running
swift --version
in your terminal, which should display the current Swift version [15].
By following these steps and utilizing the resources provided, beginners can effectively start their Swift programming journey, laying a strong foundation for more advanced topics and app development.
Fundamentals of Swift Programming
To dive deeper into Swift programming, understanding its fundamentals is crucial. Here’s a structured approach to grasp the core concepts:
Swift Programming Basics
- Data Types and Variables: Swift’s data types include Integer, Floating-Point Numbers, and Strings. Learn to declare variables (
var
) for mutable objects and constants (let
) for immutable objects. Swift ensures type safety and supports Unicode-correct strings, including emoji [10] [11]. - Control Flow: Master decision-making with if and switch statements, and iterate with loops. Swift’s control flow mechanisms are essential for directing the program’s execution flow [11].
- Functions and Collections: Functions in Swift are versatile, supporting parameters and return values. Collections, such as arrays, sets, and dictionaries, are fundamental for storing and managing groups of data. Practice creating and manipulating these to understand Swift’s powerful collection handling [11].
Advanced Concepts
- Error Handling and Optionals: Swift introduces a robust error handling model that allows developers to catch and handle errors gracefully. Understanding optionals is key to dealing with the absence of a value without causing runtime errors [11].
- Structs, Classes, and Protocols: Dive into object-oriented programming with Swift’s classes and structs, and learn how to define properties, methods, and initializers. Explore protocol-oriented programming to leverage Swift’s powerful type system and inheritance [11].
Practical Application
- Build a Single View Application: Start with a simple project to apply what you’ve learned. Use Xcode to create a single view application, focusing on layout and basic user interaction [2].
- Explore Swift 4 Features: Swift 4 introduces improved standard library, serialization, and smart key paths. It unifies procedural and object-oriented parts of the language, offering a safer and more expressive coding experience [2].
By systematically covering these fundamentals, beginners will be well-equipped to tackle more complex Swift programming challenges and start building their first applications.
Building Your First App with Swift
Building your first app with Swift is an exciting journey into app development, blending creativity with technical skills. Here’s how to embark on creating your initial applications using Swift and Xcode:
- Simple Music App with SwiftUI:
- HelloWorld App:
- Basics with Storyboard: Utilize Swift and Storyboard to craft the ‘HelloWorld’ app, a staple for beginners. This project will introduce you to Auto Layout, Stack Views, and Navigation Controller, among other foundational concepts [22].
- Basics with Storyboard: Utilize Swift and Storyboard to craft the ‘HelloWorld’ app, a staple for beginners. This project will introduce you to Auto Layout, Stack Views, and Navigation Controller, among other foundational concepts [22].
- Random Photo Generator:
- UI Components: Design your app to include a button and an image view. This simple structure is perfect for beginners [23].
- Functionality: Implement the
didTapButton()
method. When the button is pressed, this method should call thegetRandomPhoto()
function to display a new random image, showcasing how to add dynamic content to your apps [23].
- Building with Both SwiftUI and UIKit:
- SwiftUI Essentials: Explore SwiftUI for views, navigation, data passing, state management, and more. This is crucial for modern iOS app development [24].
- UIKit Fundamentals: Don’t forget about UIKit. Dive into collection views, editable views, and managing reminders to gain a comprehensive understanding of app development [24].
- First-Time User Tutorial:
- User Guidance: Implement a tutorial for new users of your app using NSUserDefaults or third-party libraries like BWWalkthrough and OnboardingKit. This step is essential for enhancing user experience and retention [25].
By following these steps, you will not only learn the Swift programming language but also gain hands-on experience in developing applications with both SwiftUI and UIKit. This practical approach ensures you’re well-equipped to tackle more complex projects in the future.
- User Guidance: Implement a tutorial for new users of your app using NSUserDefaults or third-party libraries like BWWalkthrough and OnboardingKit. This step is essential for enhancing user experience and retention [25].
Advanced Swift Programming Techniques
Diving into advanced Swift programming techniques opens up a world of possibilities for developers looking to enhance their applications and codebase. This section explores several sophisticated programming concepts and practices.
- Generics and Protocol-oriented Programming:
- Error Handling and Optionals:
- Swift’s error handling enables graceful response to runtime errors. Syntax for defining an error:
enum PrinterError: Error { case outOfPaper }
and using it:func send(job: Int) throws -> String
[26]. - Optionals are used to handle the absence of a value. Syntax:
var optionalString: String? = "An optional string."
[26].
- Swift’s error handling enables graceful response to runtime errors. Syntax for defining an error:
- Functional Programming and Extensions:
- Swift supports functional programming features like higher-order functions. Example: using
map
to square numbers:let squaredNumbers = numbers.map { $0 * $0 }
[26]. - Extensions allow adding new functionality to existing types. Syntax:
extension Double { var radians: Double { return self * .pi / 180 } }
[26].
Incorporating these techniques can significantly improve code safety, readability, and efficiency. Additionally, tools like SwiftLint help enforce coding standards for a more consistent and error-free codebase [27]. Understanding and applying these advanced concepts will elevate your Swift programming skills and open up new avenues in application development.
- Swift supports functional programming features like higher-order functions. Example: using
Conclusion
Throughout this comprehensive guide, we have explored the foundational elements of Swift programming, starting from its key features and installation steps to diving into the basics, building your first app, and finally scaling to more advanced programming techniques. We’ve seen how Swift’s design, focused on safety and speed, alongside its interoperability and open-source community, makes it a versatile and powerful choice for both new and experienced developers. By taking a step-by-step approach, readers are equipped to embark on their coding journey with confidence, leveraging Swift’s capabilities to create impactful applications.
As you continue to explore and experiment with Swift, remember that the journey of learning and development is ongoing. The practical experiences shared, from constructing basic applications to understanding advanced concepts such as generics and functional programming, serve as a solid foundation. However, the vast landscape of Swift programming is ever-evolving. Staying curious, embracing challenges, and pursuing further research and projects will not only enhance your skills but also contribute to the vibrant Swift community. With the tools and knowledge at your disposal, the potential to innovate and create with Swift is limitless.
FAQs
Q: What’s the simplest approach to mastering Swift programming?
A: The simplest approach to learning Swift, regardless of your prior coding experience, is through hands-on practice. Apple’s Swift Playground is an excellent tool for beginners to enhance their coding skills. Engaging in courses and bootcamps can also be very beneficial, as they offer the chance to work on real-world projects, accumulate a portfolio, and obtain certifications.
Q: Is Swift a suitable language for a beginner programmer?
A: Absolutely, Swift is an ideal choice for anyone looking to dive into programming, including students and individuals considering a career change. Swift was specifically created to serve as a first programming language, making it an accessible entry point into the coding world.
Q: Does learning Swift provide a good foundation for coding?
A: Yes, Swift is a fantastic language for learning to code. It has brought about a fresh approach to working with Objective-C and has made numerous enhancements to its standard library. Swift is not only easy to learn and use but also highly sought after by major technology companies, making it a valuable language to know.
Q: Can I teach myself Swift without formal instruction?
A: Indeed, self-learning can be an effective method, and Swift Playgrounds offers a playful and imaginative environment to do just that. Along with the structured “Get Started with Code” lessons, Swift Playgrounds presents a variety of challenges that invite you to continuously engage and try new things, making it a resourceful platform for independent learners.
References
[1] – https://www.geeksforgeeks.org/introduction-to-swift-programming/
[2] – https://www.javatpoint.com/swift-introduction
[3] – https://www.knowledgehut.com/tutorials/swift-tutorial/swift-overview
[4] – https://en.wikipedia.org/wiki/Swift_(programming_language)
[5] – https://www.coursera.org/articles/programming-in-swift
[6] – https://www.freecodecamp.org/news/the-swift-handbook/
[7] – https://forums.swift.org/t/is-swift-a-good-language-for-a-beginner-to-learn/59814
[8] – https://www.freecodecamp.org/news/learn-swift-basics-in-5-minutes-30a530e23231/
[9] – https://www.educative.io/blog/swift-programming
[10] – https://developer.apple.com/swift/
[11] – https://www.geeksforgeeks.org/swift-tutorial/
[12] – https://developer.apple.com/swift-playgrounds/
[13] – https://www.youtube.com/watch?v=Ulp1Kimblg0
[14] – https://forums.developer.apple.com/forums/thread/85307
[15] – https://swift.org/getting-started/
[16] – https://docs.swift.org/swift-book/LanguageGuide/TheBasics.html
[17] – https://www.codecademy.com/learn/learn-swift
[18] – https://www.youtube.com/watch?v=CwA1VWP0Ldw
[19] – https://www.programiz.com/swift-programming
[20] – https://medium.com/swift-programming/swiftui-you-got-this-learn-to-build-your-first-app-part-1-of-3-56c8b918dc0a
[21] – https://www.instructables.com/Make-an-iOS-App-with-Swift/
[22] – https://www.appcoda.com/learnuikit/build-your-first-app.html
[23] – https://www.youtube.com/watch?v=yuo50-TiKgo
[24] – https://developer.apple.com/tutorials/app-dev-training/
[25] – https://stackoverflow.com/questions/39015078/create-a-guide-tutorial-for-my-ios-app
[26] – https://clouddevs.com/swift/advanced-techniques/
[27] – https://reintech.io/blog/mastering-swift-tips-tricks-best-practices