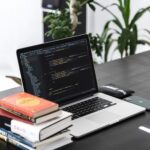
Unveiling the Power of Object-Oriented Programming in Swift: Crafting Structured and Scalable Code
March 18, 2024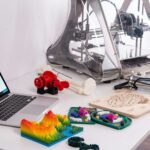
Swift Collections and Data Structures
March 19, 2024In the riveting world of mobile application development, Swift stands as a beacon of simplicity and power, yet beneath its unassuming exterior lies complexity that can trip up even seasoned coders. The abstract concepts of error and exception handling top that list. They’re the invisible safety nets that prevent minor bugs from spiraling into catastrophic system failures. You’ve got questions; we’ve got answers. ‘Error handling and exception handling in Swift’ explores these complex but fundamental aspects of Swift programming in an easy-to-understand and logical approach. It unravels the onion-like layers so you can navigate through with increased confidence and agility. Whether you’re a bright-eyed rookie or a battle-hardened veteran, this dive into the heart of Swift is sure to illuminate your path forward. So strap in, we’re about to decode the enigma of error and exception handling in Swift!
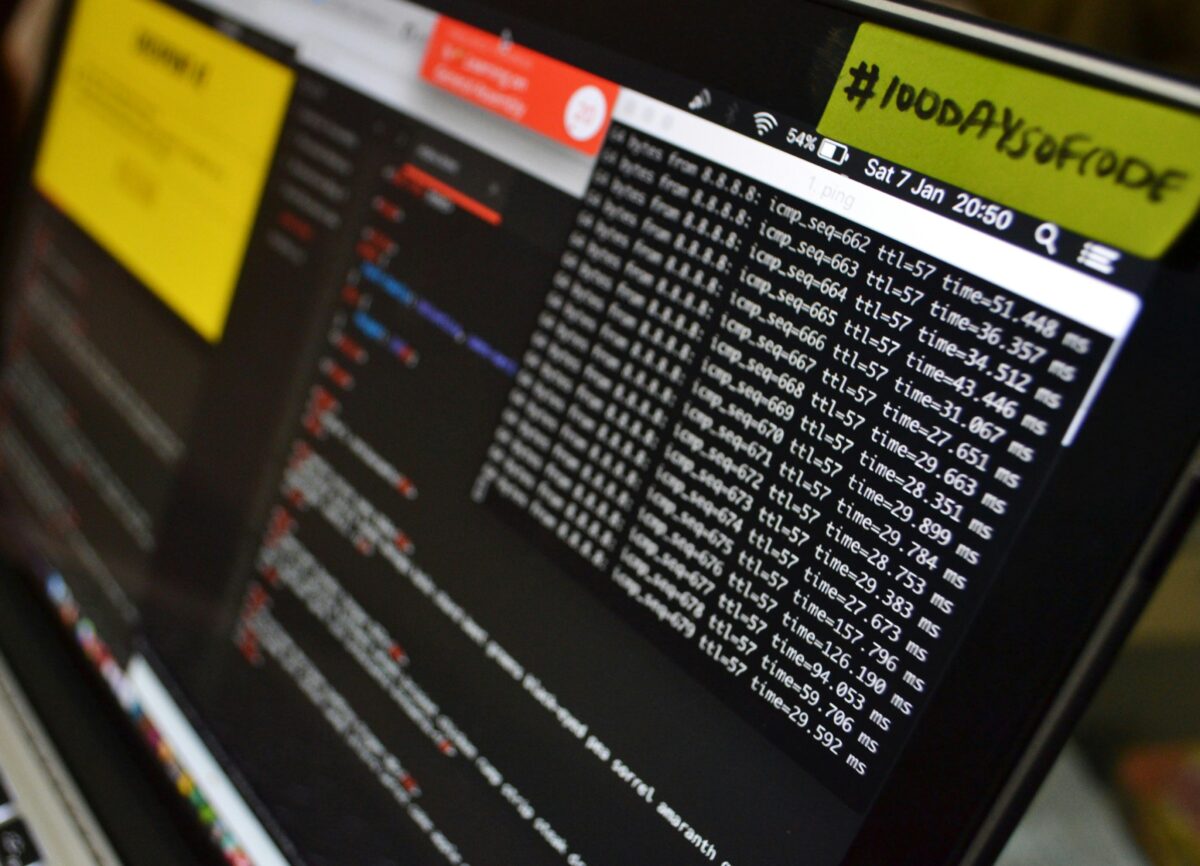
Error handling and exception handling in Swift
Table of Contents
Understanding exceptions in Swift
Swift, the intriguingly simple yet potent language for iOS application development, carries a multitude of complexities beneath its surface. Exceptions, one of the most enigmatic components, serve as an essential safety guard to ensure smooth operation. Let’s dive into the world of exceptions in Swift and understand their role and function.
Exceptions often occur when a program encounters a situation it can’t manage, causing the application to crash. In Swift, exceptions are non-local jumps in the code, which means they cause the code to leap from one point to another without following the usual sequential order. When an exception is thrown, the normal flow of the program is disrupted, and control shifts to a designated exception handler.
Contrary to some languages, Swift uses exceptions sparingly. They are generally reserved for critical programming errors that cannot be recovered from, such as out-of-bounds array indexing or nil-force-unwrapping. This selective use of exceptions in Swift is part of its design philosophy to promote safer, more predictable code.
The difference between error handling and exception handling
Though at first glance, error handling and exception handling might appear similar, they serve different purposes in Swift. Understanding the distinction between the two can help developers write more robust and reliable code.
Error handling in Swift is a process used to respond to error conditions while the program is running. Errors are represented by values of types conforming to the Error protocol. These errors are expected and recoverable, and Swift provides several ways to catch and handle them. For instance, a file-not-found error can be caught and handled by prompting the user to select a different file.
On the other hand, exception handling is a method to respond to exceptional circumstances during the runtime that are typically out of the control of the program. As mentioned before, exceptions are used for critical errors from which recovery is not possible. One common example is an out-of-bounds error when accessing an array.
Techniques for error handling in Swift
Swift offers several techniques for error handling, each with its own use case. These techniques provide developers with flexibility and control over how errors are handled in their code.
The most common method is using the do-catch
syntax. The do
block encapsulates the code that may cause an error, while the catch
block captures that error and responds appropriately. This technique allows developers to isolate error-prone code and handle errors gracefully.
Swift also provides the try?
and try!
keywords for error handling. try?
changes the result into an optional value, returning nil if an error is thrown. try!
is used when the developer is sure no error will be thrown. If an error does occur, it leads to a runtime crash.
Techniques for exception handling in Swift
Exception handling in Swift is a bit more complex than error handling. Given that Swift doesn’t support traditional exception handling mechanisms like try-catch-throw
, we have to rely on other techniques.
One approach is using the Objective-C interoperability of Swift. Objective-C has a full-featured exception handling mechanism, and Swift can leverage this when calling Objective-C code. The @objc
attribute can be used to expose Swift methods to Objective-C, which can then throw exceptions.
Another technique is using error pointers. Some APIs provide an extra error pointer parameter that gets filled if an error occurs. This technique can be useful when dealing with APIs that throw exceptions and can be used to convert exceptions into Swift errors.
Best practices for error and exception handling in Swift
Good error and exception handling can make the difference between a frustrating or pleasant user experience. Here are some best practices to ensure your Swift applications are resilient and user-friendly.
Ensure to encapsulate all error-prone code within a do-catch
block. This practice enhances the reliability of your program and prevents unexpected crashes. Additionally, when using try?
or try!
, be aware of the potential implications. try!
should only be used when you’re certain no error will be thrown.
For exception handling, remember that Swift doesn’t provide built-in support for exceptions. As such, exceptions should be reserved for programming errors, not for control flow. Also, avoid using exceptions for expected or recoverable errors.
Common errors in Swift and how to handle them
Swift applications can encounter a range of errors during their lifecycle. Here are some common errors and how to handle them.
A common error is the fileNotFound
error. This error occurs when a file that the program is trying to access does not exist. To handle this error, you can use a do-catch
block to catch the error and inform the user.
Another common error is the invalidArgument
error, which occurs when an invalid argument is passed to a function. You can catch this error using a do-catch
block and provide feedback to the user or developer.
Common exceptions in Swift and how to handle them
While exceptions are less common in Swift, they can still occur and cause havoc if not properly managed. Two common exceptions are outOfBounds
and unexpectedNil
.
The outOfBounds
exception occurs when an attempt is made to access an array element that is out of its bounds. This exception can be avoided by always checking the array’s count before accessing its elements.
The unexpectedNil
exception occurs when an optional value is force-unwrapped while it contains a nil value. This exception can be avoided by always using optional binding or optional chaining before force-unwrapping an optional.
Tools for debugging errors and exceptions in Swift
Debugging is an essential part of programming, and Swift provides several tools to aid in this process. The Xcode debugger and LLDB are two such tools that can be used to inspect and diagnose errors and exceptions.
The Xcode debugger provides a visual interface for debugging Swift code. It allows developers to set breakpoints, step through code, and inspect variable values.
LLDB, on the other hand, is a command-line debugger that provides powerful scripting and extension capabilities. It can be used to make complex queries and manipulations that would not be possible with the Xcode debugger.
Conclusion
Understanding and properly handling errors and exceptions are fundamental to developing robust and reliable Swift applications. By following best practices and using the right techniques, developers can ensure their applications are resilient and offer a smooth user experience.
Swift’s unique approach to error and exception handling, coupled with its strong typing system, encourages developers to write safer and more predictable code. By leveraging the tools and techniques discussed in this article, you can navigate the complexities of Swift with increased confidence and agility.