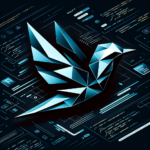
Getting Started with Swift
March 16, 2024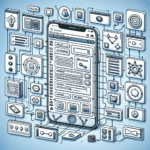
Understanding UIKit for iOS Development
March 16, 2024Are you eager to build your very first iOS app using Swift? Look no further! In this comprehensive guide, we will walk you through the process step by step, ensuring that you have all the knowledge and tools needed to create your own app. Whether you’re a seasoned programmer or a beginner, this article is designed to help you. With its clean and easy-to-read syntax, Swift has become the go-to programming language for iOS development. We will explore the basics of Swift, from variables and functions to loops and conditionals. You will also learn how to design your app’s user interface using the powerful UIKit framework. But we won’t stop there! We will delve into more advanced topics such as handling user input, connecting to web services, and storing data locally. By the end of this article, you will have a solid understanding of iOS app development and be well on your way to building your first app. So, let’s jump in and start building together! Get ready to unleash your creativity and bring your app ideas to life.
Building Your First iOS App using Swift
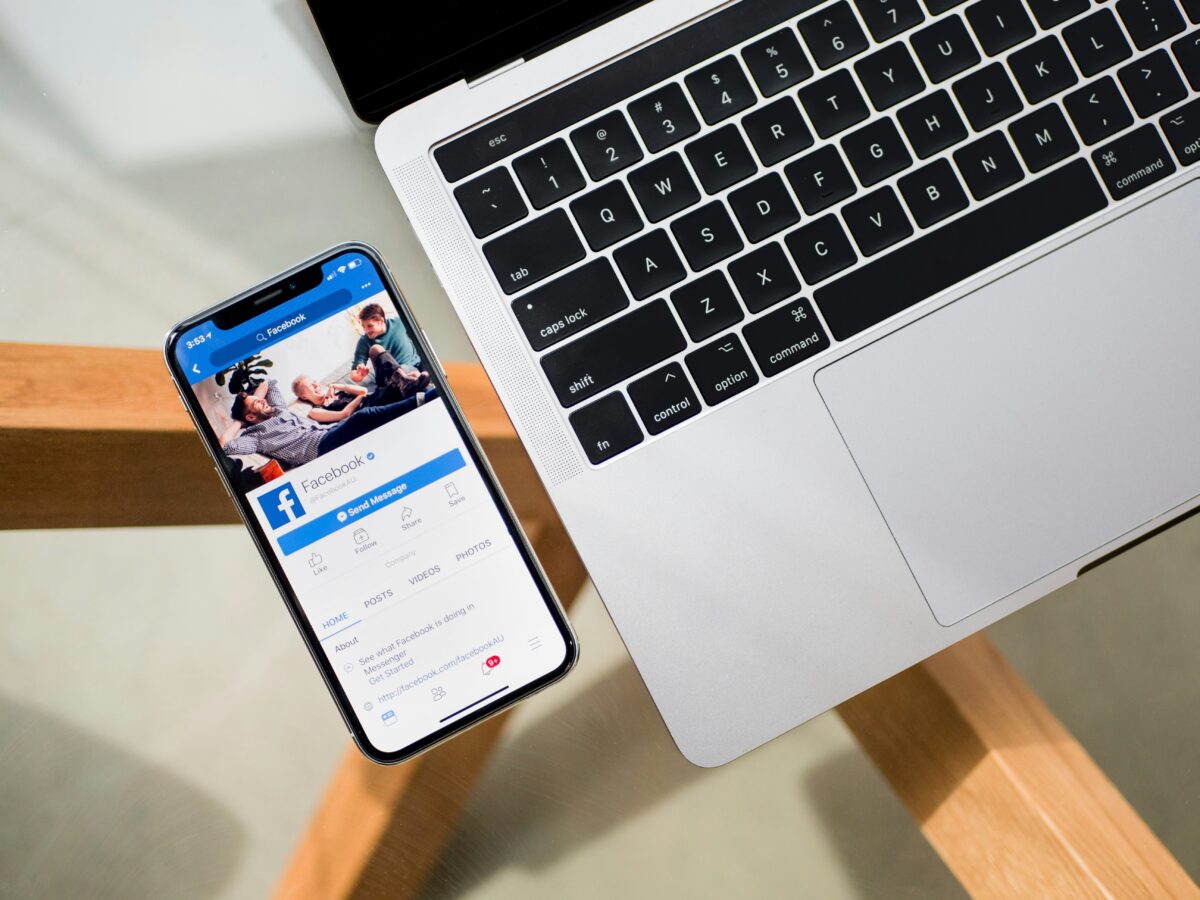
Introduction
Are you eager to build your very first iOS app using Swift? Look no further! In this comprehensive guide, we will walk you through the process step by step, ensuring that you have all the knowledge and tools needed to create your own app. Whether you’re a seasoned programmer or a beginner, this article is designed to help you.
With its clean and easy-to-read syntax, Swift has become the go-to programming language for iOS development. We will explore the basics of Swift, from variables and functions to loops and conditionals. You will also learn how to design your app’s user interface using the powerful UIKit framework.
But we won’t stop there! We will delve into more advanced topics such as handling user input, connecting to web services, and storing data locally. By the end of this article, you will have a solid understanding of iOS app development and be well on your way to building your first app. So, let’s jump in and start building together! Get ready to unleash your creativity and bring your app ideas to life.
Understanding the Swift Programming Language
Swift is a powerful, easy-to-learn programming language developed by Apple. It is the primary language for iOS, macOS, watchOS, and tvOS app development. Swift was designed to be safe, fast, and easy to read and write. It offers modern programming features like optionals, closures, and generics, making it a great choice for app development.
Swift uses a clean syntax that is easy to read and write. It eliminates entire classes of unsafe code like null pointers, and its memory management is handled automatically, so you don’t have to worry about memory leaks. Swift also includes modern features like dynamic libraries, which can be updated independently of the rest of the app, reducing the size of your apps and improving their performance.
Swift also integrates seamlessly with Objective-C, which means you can use Swift and Objective-C code in the same project. This makes it much easier to adopt Swift in your existing projects, and it provides a path to gradually transition your codebase to Swift.
Setting Up the Development Environment

Before you can start programming in Swift, you need to set up your development environment. The main tool you’ll need is Xcode, which is Apple’s integrated development environment (IDE) for macOS. Xcode provides everything you need to create apps for Apple’s platforms, including a source editor, a graphical user interface editor, and a debugger.
You can download Xcode for free from the Mac App Store. Once you’ve installed Xcode, you’ll also have access to the Swift Playgrounds feature, which is an interactive way to learn and experiment with Swift. Playgrounds are a great way to practice Swift coding and see the results of your code immediately, without having to create a full app project.
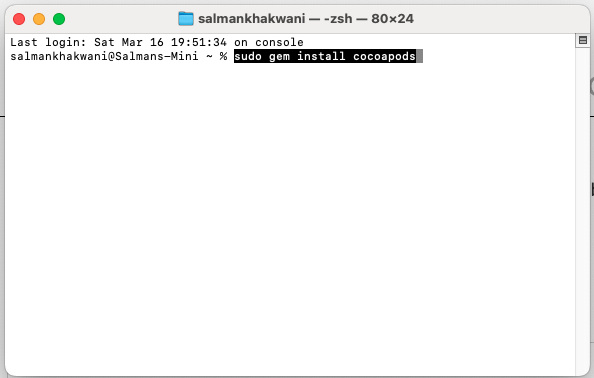
In addition to Xcode, you might also find it useful to install some other tools that can help you with your Swift development. For example, GitHub is a popular platform for version control and collaboration, and Cocoapods is a helpful tool for managing third-party libraries in your projects.
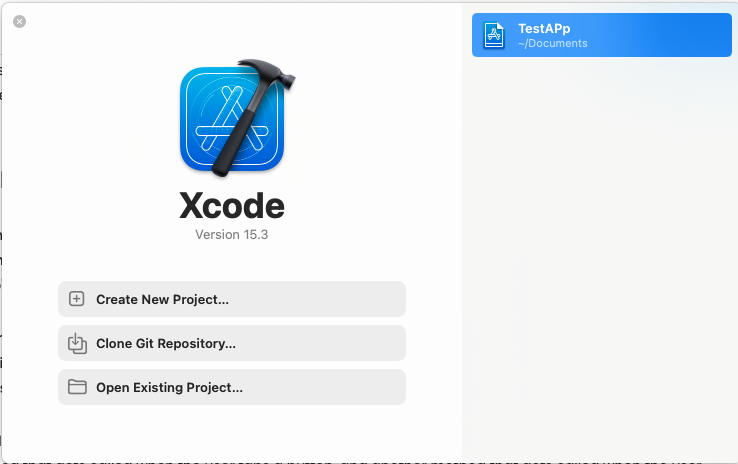
Creating a New Xcode Project
Once you’ve set up your development environment, the next step is to create a new Xcode project. This is where you’ll do all your coding and design work. To create a new project, open Xcode and select “Create a new Xcode project” from the welcome screen, or select “New” from the “File” menu.
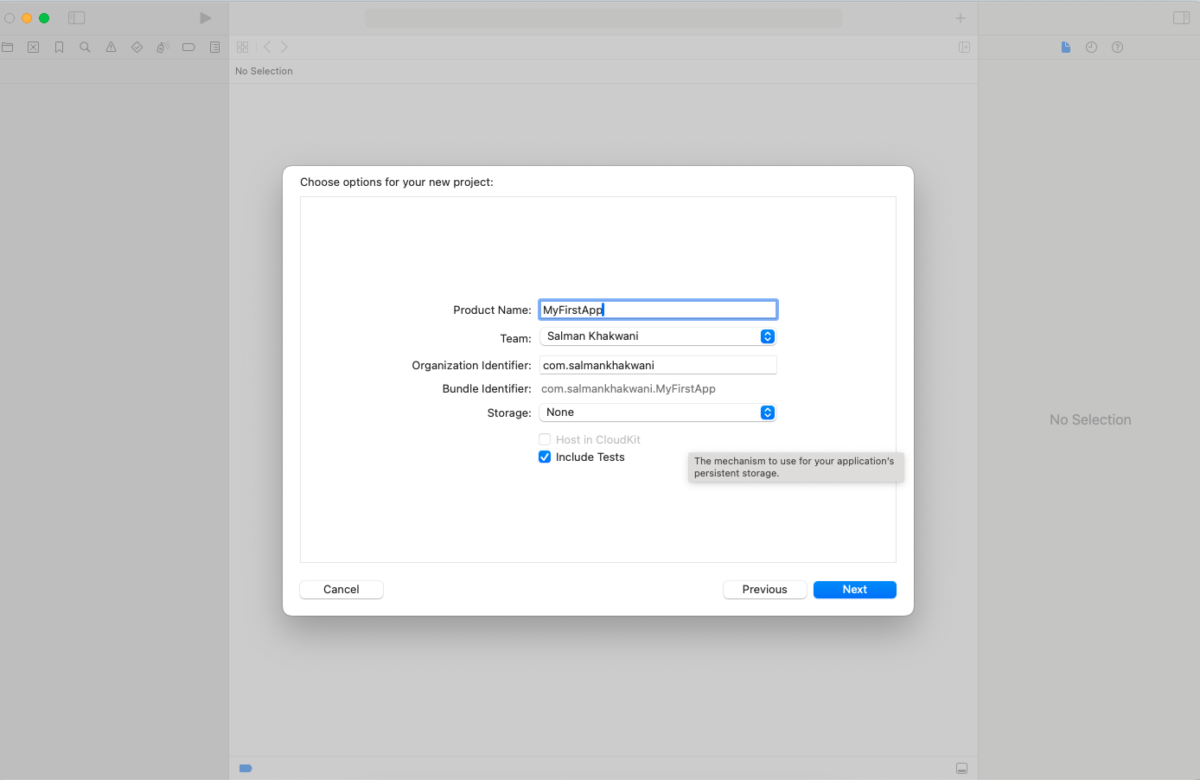
Xcode will guide you through the process of setting up your project. You’ll need to choose a template for your project – for iOS apps, you’ll usually choose the “Single View App” template. You’ll also need to provide some information about your app, like its name and the organization that’s developing it. You can also specify whether you want to include unit tests or UI tests in your project.
Once you’ve created your project, Xcode will open the project editor. This is where you can configure your project’s settings, add new files and resources, and manage your project’s schemes and targets. It’s also where you’ll write your Swift code.
Designing the User Interface with Interface Builder
Interface Builder is a powerful tool that’s part of Xcode, and it allows you to design your app’s user interface visually. You can drag and drop elements like buttons, labels, and text fields onto your app’s screens, and you can adjust their properties and layout. This lets you design your app’s interface without having to write any code.
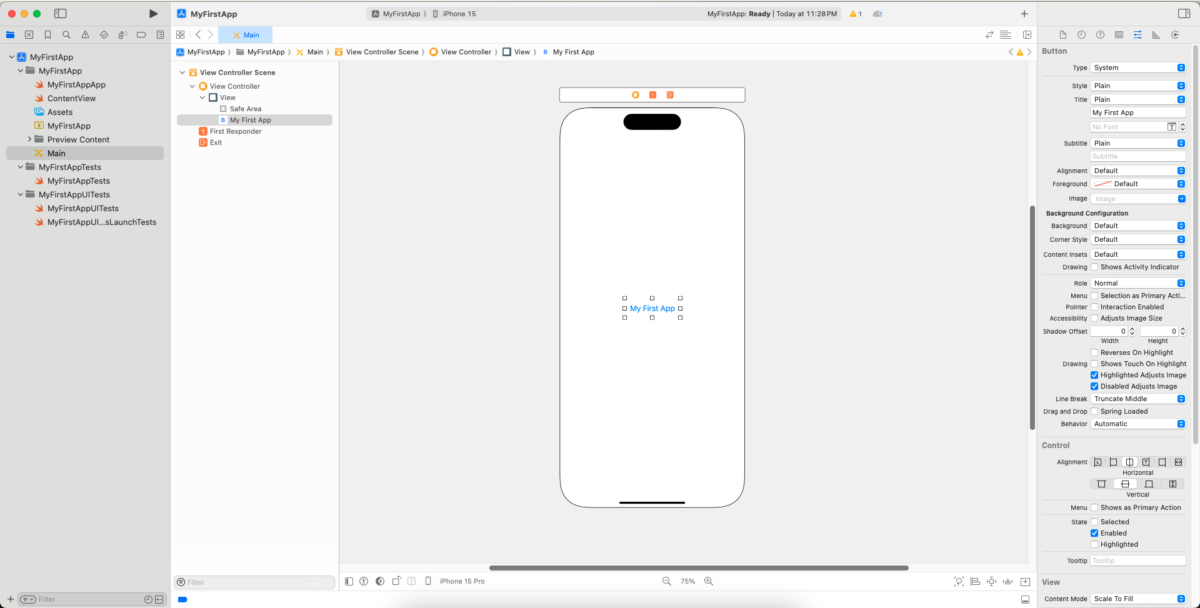
To use Interface Builder, open the storyboard file for your app’s screen (usually named “Main.storyboard”) in Xcode. You’ll see a visual representation of your screen, and you can add, remove, and modify elements using the inspector panel on the right.
Interface Builder also lets you create connections between your interface and your code. For example, you can connect a button in your interface to a method in your code that gets called when the button is tapped. These connections are called “outlets” and “actions”, and they’re a key part of making your app interactive.
Implementing Functionality with Swift Code
Once you’ve designed your app’s interface, the next step is to write the Swift code that implements your app’s functionality. This involves defining classes, functions, and variables, and writing code that responds to user actions and manages your app’s data.
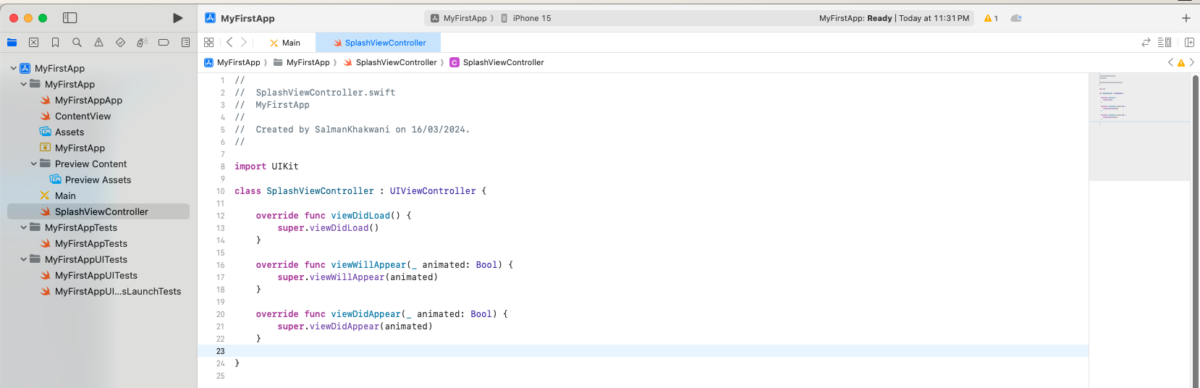
Your code will be organized into different files and groups in Xcode. The main file for each screen of your app is called a “view controller”. This file includes a class that inherits from UIViewController
or one of its subclasses. The view controller is responsible for managing the screen’s layout and responding to user actions.
In your view controller, you can define methods that get called when the user interacts with your app. For example, you might have a method that gets called when the user taps a button, and another method that gets called when the user enters text into a text field. These methods can update your app’s data, perform actions like navigating to another screen, or interact with system services like the camera or the network.
Testing and Debugging Your iOS App
Testing is a crucial part of the app development process. It ensures that your app works as expected and that it’s free of bugs. Xcode includes a suite of tools that make it easy to test your app at every stage of development.
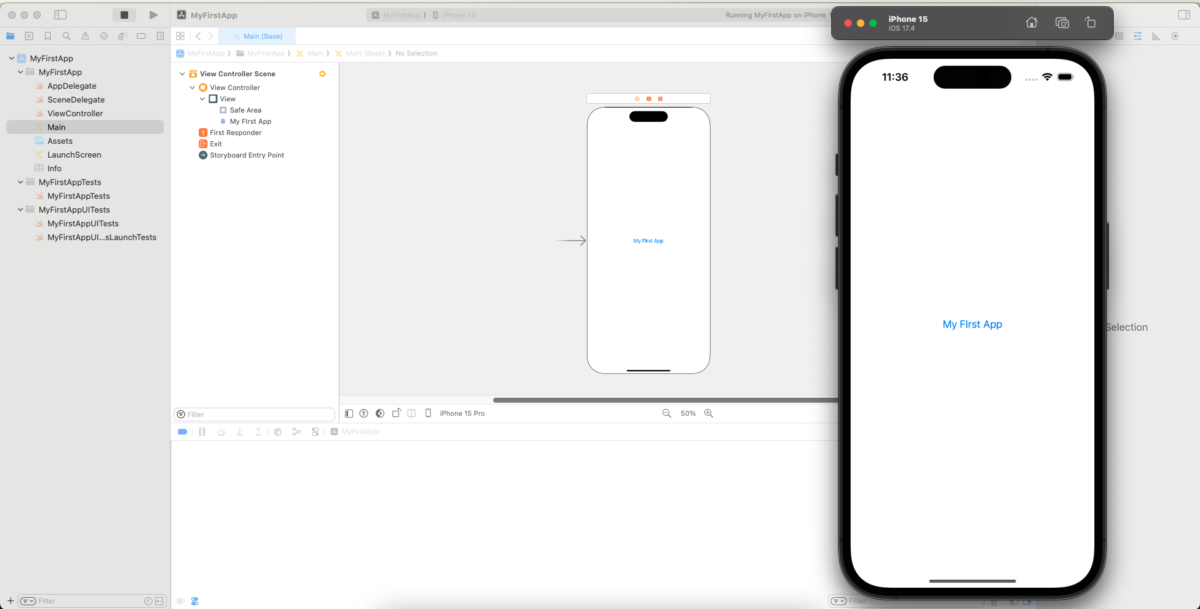
The simplest way to test your app is to run it in the simulator, which is a program that mimics the behavior of an iOS device. You can interact with your app in the simulator just like you would on a real device, and you can use the simulator to test your app on different device models and screen sizes.
In addition to manual testing, Xcode also supports automated testing. You can write unit tests and UI tests that verify your app’s behavior automatically. This is a great way to catch bugs early and ensure that your app stays reliable as you add new features and make changes.
Deploying Your App to the App Store
Once you’re happy with your app, the final step is to deploy it to the App Store. This involves preparing your app for release, submitting it to Apple for review, and managing your app’s listing on the App Store.
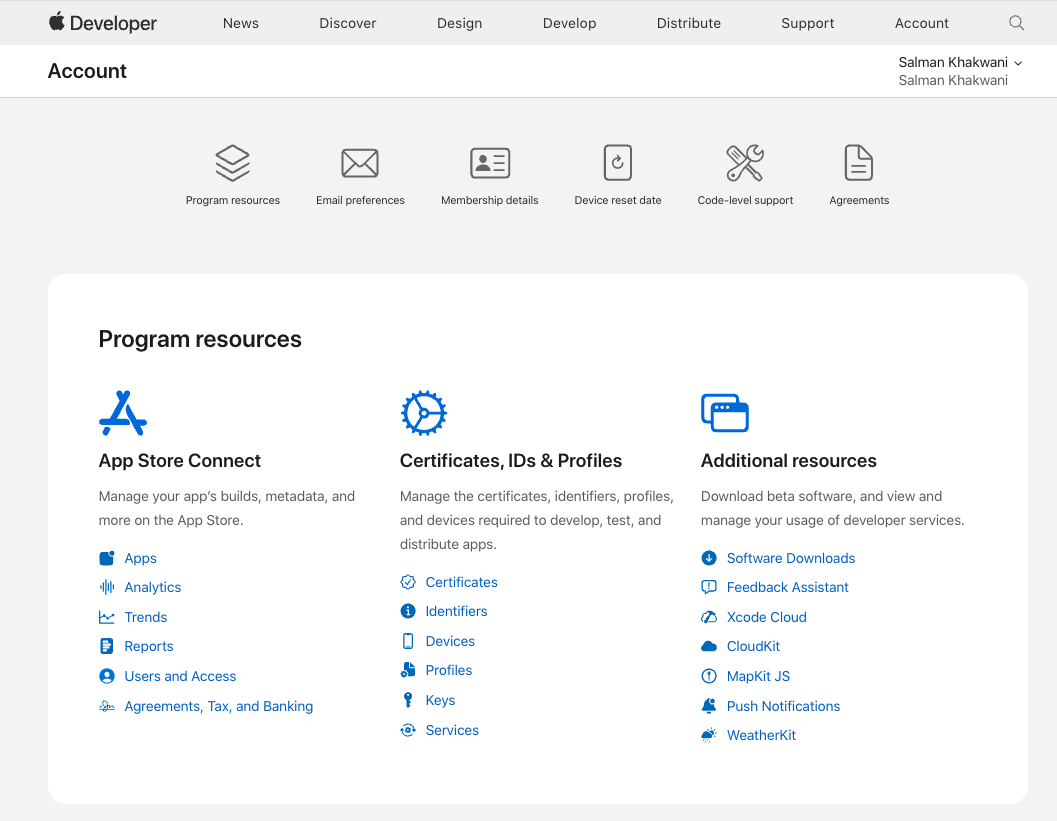
Before you can submit your app, you’ll need to enroll in the Apple Developer Program, which costs $99 per year. This gives you access to the tools and resources you need to distribute your app, and it lets you submit apps to the App Store and other Apple platforms.
Submitting your app to the App Store is a multi-step process that involves creating an App Store Connect account, configuring your app’s metadata and screenshots, building an archive of your app, and uploading it to App Store Connect. Once you’ve submitted your app, you’ll need to wait for Apple’s review team to approve it before it can go live on the App Store.
Resources for Further Learning and Support
Building an app is a big task, but it’s also a great opportunity to learn and grow as a developer. There are many resources available to help you along the way, from online tutorials and courses, to books, forums, and developer communities.
Apple’s own documentation and developer resources are a great place to start. The Swift Programming Language Guide is a comprehensive resource that covers all aspects of the language, and the iOS Developer Library includes detailed guides and sample code for all of Apple’s frameworks and services.
There are also many online platforms like Swift Playgrounds, Codecademy, Udacity, and Coursera that offer Swift and iOS development courses. These platforms cater to different learning styles and levels of experience, so you can find the one that suits you best.
Conclusion
Building your first iOS app using Swift is a significant achievement. It takes time, patience, and a lot of learning, but the result is a product that you can be proud of. Whether you’re looking to become a professional iOS developer, or you just want to create an app for fun, the skills and experience you gain from this process are invaluable.
Remember, the journey of building an app is not just about the end product. It’s also about the skills you develop, the problems you solve, and the knowledge you gain along the way. So don’t be afraid to dive in, make mistakes, and learn as you go. Happy coding!